Introduction to Common Mistakes in JavaScript Programming
JavaScript is one of the most widely used programming languages for web development. Whether you’re new to coding or have been developing for years, avoiding common mistakes is crucial for writing clean, efficient, and error-free code. In this article, we’ll walk you through the typical mistakes developers make in JavaScript and offer tips on how to avoid them. By knowing these pitfalls, you can code more effectively, save time, and avoid frustration.
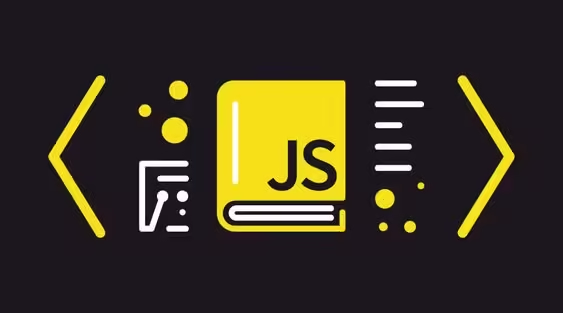
1: Not Understanding the Basics of JavaScript Syntax and Structure
Starting off strong means understanding JavaScript’s syntax and structure. Skipping this step leads to confusion later on. JavaScript has unique characteristics, such as semicolons, curly braces, and variable declarations, that might seem tricky at first but are essential to learn.
Why This Mistake Happens
Many beginners dive into advanced topics too soon, overlooking fundamental concepts, which leads to errors even in simple operations.
How to Avoid It
Focus on mastering the basics of JavaScript. Take the time to fully understand data types, operators, and control structures. Writing small, simple programs is a great way to solidify these concepts before moving on to larger projects.
For more details on JavaScript syntax, check out Mozilla’s JavaScript Guide.
2: Overcomplicating Code Instead of Keeping It Simple and Readable
Complex code might seem impressive, but it often results in code that’s hard to debug or maintain. Keeping your code clean and straightforward should always be a priority.
Why This Mistake Happens
Sometimes developers over-engineer solutions or try to use advanced logic, making the code unnecessarily complicated.
How to Avoid It
Follow the KISS (Keep It Simple, Stupid) principle. Break problems into smaller parts, use clear variable names, and avoid overly complex solutions. If your code is easy to read, it will be easier to debug later.
Learn more about writing clean code from Clean Code by Robert C. Martin.
3: Ignoring Error Handling and Skipping Testing
Errors are inevitable in coding. One of the most common mistakes is failing to handle these errors effectively or neglecting to test your code.
Why This Mistake Happens
Developers sometimes skip error handling or testing to save time, thinking they’ll return to it later.
How to Avoid It
Make error handling part of your routine. Use try...catch
blocks and test your code in various scenarios. There are great tools like Jest or Mocha that can help with automated testing.
4: Using Global Variables Instead of Local Variables
Global variables can cause unintended interactions and make debugging a nightmare. Overusing them is a common mistake.
Why This Mistake Happens
Global variables make it easy to access data anywhere in the code, but they can lead to scope issues and name conflicts.
How to Avoid It
Stick to local variables whenever possible. Local variables reduce the risk of conflicts and are easier to manage. Use let
and const
to control scope effectively.
Read more on scoping and variables in JavaScript Scoping and Hoisting.
5: Neglecting to Optimize Code for Performance
Code that works doesn’t always mean it’s optimized. Neglecting performance can slow down your app, especially in larger projects.
Why This Mistake Happens
Many developers are focused on functionality and overlook performance optimization.
How to Avoid It
After your code works, spend some time optimizing it. Techniques like debouncing, throttling, and lazy loading can significantly improve performance. Minimize redundant computations and large loops.
See Google’s Web Dev Guide for performance optimization tips.
6: Relying Too Much on Libraries
Libraries like jQuery or React can simplify your life, but relying on them without understanding JavaScript itself can limit your problem-solving skills.
Why This Mistake Happens
Developers sometimes use libraries as a shortcut to avoid learning JavaScript’s more complex features.
How to Avoid It
Learn JavaScript basics first, then use libraries to make your life easier. Understanding the underlying code will help you make better decisions when using external tools.
Explore more in You Don’t Know JS by Kyle Simpson for deep JavaScript understanding.
7: Failing to Keep Up with JavaScript Updates
JavaScript is constantly evolving, and failing to keep up can result in outdated or inefficient code. Sticking with old practices may limit your code’s performance and security.
Why This Mistake Happens
It’s easy to get comfortable with familiar methods and overlook new updates or best practices.
How to Avoid It
Keep learning! Follow blogs, join developer communities, or check out platforms like JavaScript Weekly for regular updates.
8: Not Commenting Code Properly
Comments are key to maintaining your code and making it understandable for others. Without proper comments, future developers (or you) may struggle to figure out what your code is doing.
Why This Mistake Happens
Some developers think their code is self-explanatory, skipping comments altogether.
How to Avoid It
Use concise comments to explain important parts of your code. Good comments clarify logic and can save you or others a lot of time down the line.
9: Forgetting to Use Strict Mode
Strict mode in JavaScript helps identify common coding mistakes early. Not using strict mode can lead to hard-to-find bugs.
Why This Mistake Happens
Some developers don’t know about strict mode or forget to use it in their projects.
How to Avoid It
At the start of your JavaScript files, add 'use strict';
to catch common errors early and improve code security.
Read more on Strict Mode in JavaScript.
Conclusion
Writing JavaScript without errors is tough, but being aware of common mistakes can help you avoid them. Master the basics, simplify your code, handle errors properly, and stay current with updates. By following these tips, you’ll write better, cleaner, and more efficient code.
More From Us:
7 Programming Languages You Must Master (and a Few You Might Want to Avoid) in 2024 and Beyond
Exciting Evolution of Web Development from 1990s: Overcoming Challenges Through Time
FAQs
1. Why should I avoid global variables?
Global variables can cause name collisions and are harder to debug. Use local variables to avoid conflicts and make your code more maintainable.
2. What is strict mode in JavaScript, and why should I use it?
Strict mode catches common mistakes and makes your code more secure by preventing errors like using undeclared variables.
3. How can I optimize JavaScript for performance?
To optimize performance, minimize unnecessary loops, reduce computations, and use techniques like lazy loading and debouncing.
4. Why is commenting important?
Comments clarify your code’s purpose, making it easier to understand for others or when you revisit your code later.
5. How can I stay updated with the latest JavaScript trends?
Join online communities, follow JavaScript blogs, and subscribe to newsletters like JavaScript Weekly to stay informed about the latest trends.